Have you ever needed to include the same data on every template within a Django project? One way of achieving this is to pass the same data to the template in every view across the project. However, as your project grows, and with it the number of views, this can become a nuisance. Instead, I recommend becoming familiar with Django’s custom context processors. They’re extremely easy to set up, can save you a lot of time when working on larger projects, and keep your project DRY.
In case you are wondering what DRY means, it stands for Don’t Repeat Yourself. DRY programming is a common and encouraged programming design principle found within the Django framework and community. Adhering to DRY programming principles includes replacing redundant functionality in your code with abstractions. This can be extremely helpful in avoiding unnecessary mistakes and saving time when rewriting code. As we will see in the examples below, it may often be necessary to utilize the same or similar functionality across many parts of a project. Rather than spending the time to write out the full logic each and every time, Django offers ways to remove this logic so that we can obtain the necessary data with a simple function call. In the context of this article, we follow the DRY principle by replacing redundant logic within each view with one context processor that can be called directly from our templates.
Let’s consider a Django project that displays the current date on every page. As mentioned before, one way to include the date would be to pass the data in as context within each view.
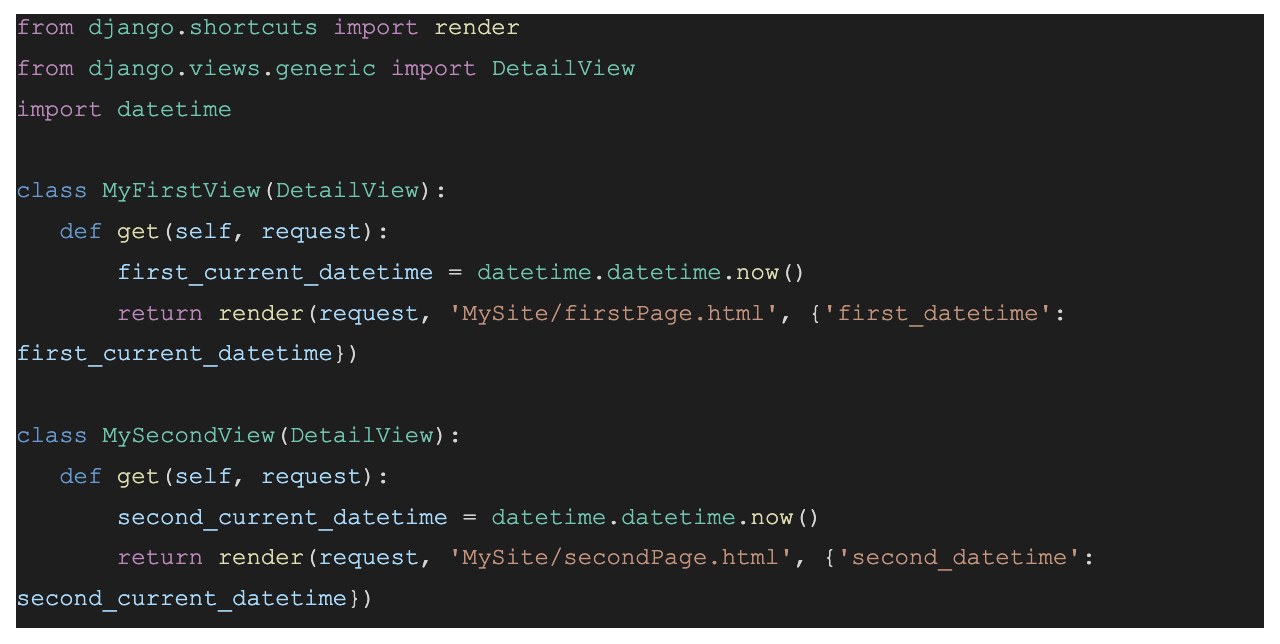
To avoid having to get the current datetime within each method on each view create a new file within your app and let’s build our own custom context processor.

As you can see, a context processor is simply a python function that takes the current request as an argument and returns a dictionary of values that you would like to have access to within the context of multiple templates.
Next, to access the previously created dictionary, add your custom context processor to the list of context processors in your settings.py file.
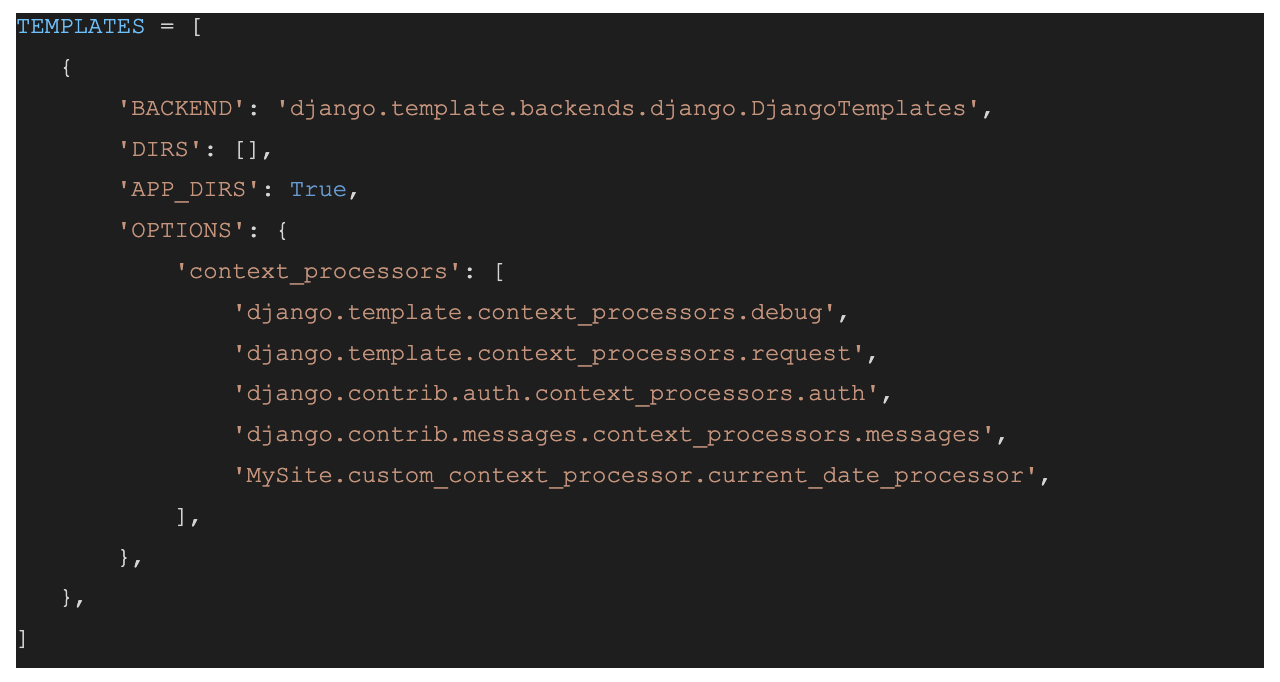
You’re good to go! Now, within any of the templates, you can access this function and with it the current date using current_datetime.

Now that you know how to set up a custom context processor you can use them to include models from the database, current user information, or just about any other data that you may need access to across multiple templates.